· yebor974 · Getting Started, Tutorials
Quick installation and configuration of a Filament panel
Install a modern admin panel with Filament PHP in minutes! Set up, configure, and customize seamlessly for your Laravel app. Start building today!
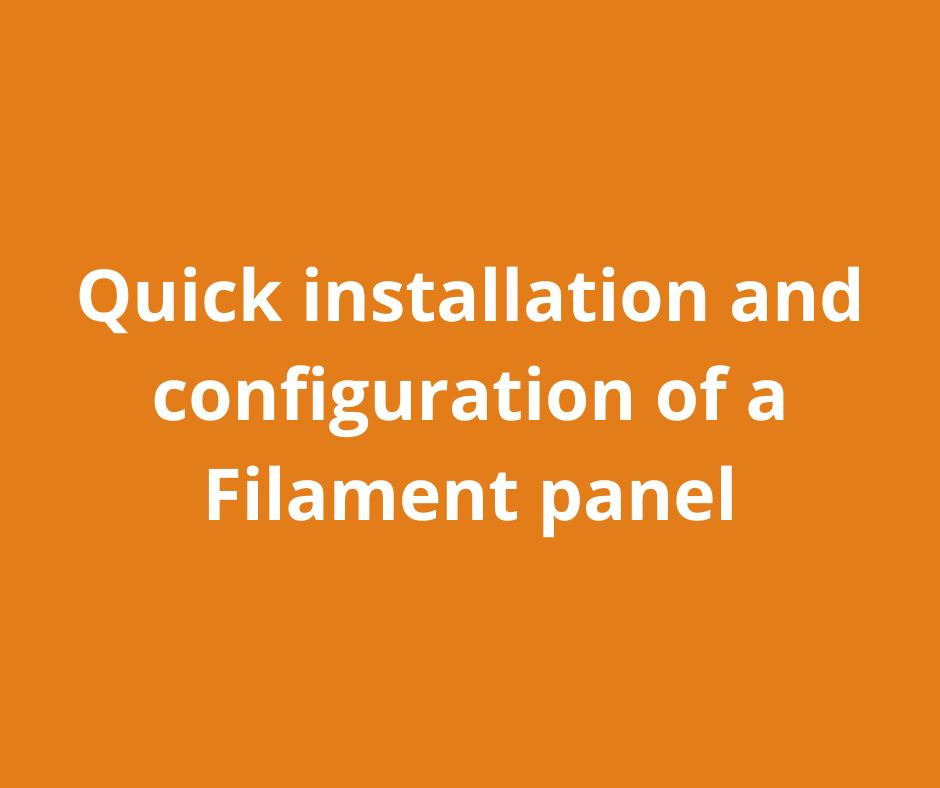
Filament PHP is a modern and powerful library that makes creating administration panels for Laravel applications seamless. This article will guide you through the steps to install Filament, configure a panel, and allow an admin user to log in.
What is a Filament panel?
A Filament panel is a user interface designed to manage your Laravel application data. It’s ideal for dashboards, CRUD tools, and advanced administrative features.
Key advantages of Filament:
- Modern and responsive interface built with Tailwind CSS.
- Quick setup and developer-friendly commands.
- Customizable and extendable with plugins and advanced configurations.
Step 1: Set Up a Laravel project
Before installing Filament, you need a working Laravel project. Create a new project using the following command:
composer create-project laravel/laravel your-project-name
This command:
- Install a new Laravel app instance
- Sets up a default
.env
config file with sqlite - Run the default migrations with tables like
users
Step 2: Install Filament PHP
To add Filament to your Laravel project, follow these steps:
-
Install the package via Composer:
composer require filament/filament:"^3.2" -W
-
Set up the Filament panel:
php artisan filament:install --panels
You’ll be prompted to enter a panel name (default: admin) and encouraged to star the Filament repository on GitHub. Go ahead and do it! 😊 This command:
- Generates a new Service Provider
app/Providers/Filament/AdminPanelProvider.php
or with your panel name alias. - Sets up panel routes (default:
/admin
). - Installs necessary CSS and JavaScript files.
Your new Service Provider looks like that :
class AdminPanelProvider extends PanelProvider
{
public function panel(Panel $panel): Panel
{
return $panel
->default()
->id('admin')
->path('admin')
->login()
->colors([
'primary' => Color::Amber,
])
->discoverResources(in: app_path('Filament/Resources'), for: 'App\\Filament\\Resources')
->discoverPages(in: app_path('Filament/Pages'), for: 'App\\Filament\\Pages')
->pages([
Pages\Dashboard::class,
])
->discoverWidgets(in: app_path('Filament/Widgets'), for: 'App\\Filament\\Widgets')
->widgets([
Widgets\AccountWidget::class,
Widgets\FilamentInfoWidget::class,
])
->middleware([
EncryptCookies::class,
AddQueuedCookiesToResponse::class,
StartSession::class,
AuthenticateSession::class,
ShareErrorsFromSession::class,
VerifyCsrfToken::class,
SubstituteBindings::class,
DisableBladeIconComponents::class,
DispatchServingFilamentEvent::class,
])
->authMiddleware([
Authenticate::class,
]);
}
}
Of course, you can create multiple panels. For example, you could have an admin panel for administration with a specific guard and a member panel for users. The Filament Mastery blog uses a similar setup with two panels. 😊
To create an other panel:
php artisan make:filament-panel your-new-id-panel
This command:
- Generates a new Service Provider
app/Providers/Filament/MemberPanelProvider.php
if your new id panel parameter is member.
If you plan to work with two or more panels, consider refactoring your default AdminPanelProvider to organize your code into subdirectories and segmented modules for better maintainability.
class AdminPanelProvider extends PanelProvider
{
public function panel(Panel $panel): Panel
{
return $panel
...
->discoverResources(in: app_path('Filament/Admin/Resources'), for: 'App\\Filament\\Admin\\Resources')
->discoverPages(in: app_path('Filament/Admin/Pages'), for: 'App\\Filament\\Admin\\Pages')
..
->discoverWidgets(in: app_path('Filament/Admin/Widgets'), for: 'App\\Filament\\Admin\\Widgets')
...
}
}
User
model
Step 3: Configure the To allow login access to the Filament panel, update the User
model as follows:
- Open
app/Models/User.php
. - Add the
FilamentUser
interface and implement thecanAccessPanel
method:
This example restricts panel access to users with a specific email domain. You can update it with your own rules or simply have it return<?php namespace App\Models; use Illuminate\Foundation\Auth\User as Authenticatable; use Filament\Models\Contracts\FilamentUser; class User extends Authenticatable implements FilamentUser { public function canAccessPanel(): bool { return str_ends_with($this->email, '@yourdomain.com'); } }
true
.
Step 4: Create an user
Run the following command to create an admin user who can log in:
php artisan make:filament-user
You’ll be prompted to enter a name, email, and password. If you prefer not to be prompted, you can pass parameters directly in the command line:
php artisan make:filament-user --name=name --email=email@domain.tdl --password=xxxxxxxxxx
Step 5: Access the panel
The setup is now complete. Start the Laravel server:
php artisan serve
Visit the panel in your browser at:
http://localhost:8000/admin
Log in using the credentials created in the previous step.
Next Steps: Customize and extend filament
With the panel running, you can:
- Build CRUD resources to manage your Laravel models.
- Customize the UI by modifying views and forms.
- Install third-party plugins for advanced features like role management and analytics.
Conclusion
Filament PHP makes it easy to integrate a modern, functional admin panel into a Laravel application. With just a few commands, you’ll have a powerful tool ready for customization.