· yebor974 · Getting Started, Tutorials
Filament Render Hooks: example with authentication pages
Learn how to customize Filament panels using Render Hooks. Discover the power of clean and maintainable customizations in Filament!
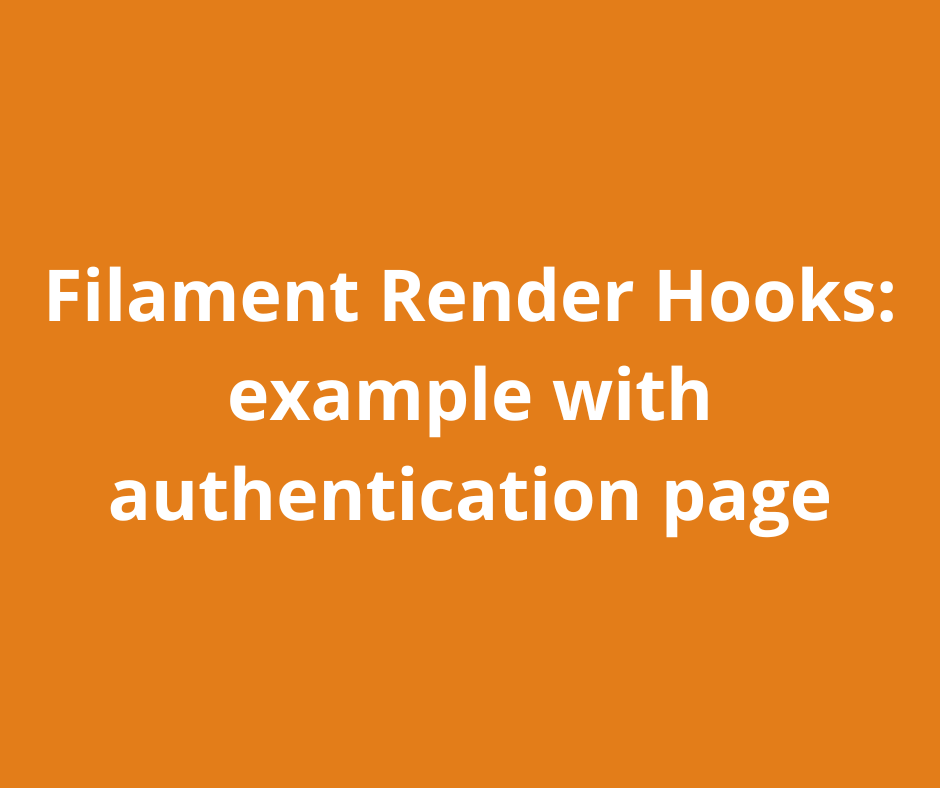
In this article, we’ll dive into an essential customization feature in Filament: Render Hooks. These hooks allow you to insert or modify HTML content in specific parts of your Filament pages without overriding or duplicating existing views. We'll demonstrate this with a practical example: adding a "Back to Website" link above the login and register forms in the admin panel.
What is a Render Hook?
A Render Hook in Filament is a predefined insertion point in the views where you can add HTML or Blade content using the FilamentView::registerRenderHook
method.
Advantages of Render Hooks:
- No need to publish or modify Filament's views.
- Reduces potential conflicts during Filament updates.
- Flexibility to inject content at strategic locations.
Practical example: adding a "Back to Website" link
Let’s explore how to add a "Back to Website" link above the login and register forms in the Filament admin panel.
This setup is added to the AppServiceProvider
within the boot()
method, ensuring the hooks are registered when the application boots. You can also use it on middleware.
namespace App\Providers;
use Filament\Facades\FilamentView;
use Filament\View\PanelsRenderHook;
use Illuminate\Support\Facades\Blade;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
public function boot(): void
{
// Add a link above the login form
FilamentView::registerRenderHook(
PanelsRenderHook::AUTH_LOGIN_FORM_BEFORE,
fn (): string => Blade::render('<x-filament::link href="' . config('app.url') . '" size="sm" icon="heroicon-o-arrow-left">Back to Website</x-filament::link>')
);
// Add a link above the register form
FilamentView::registerRenderHook(
PanelsRenderHook::AUTH_REGISTER_FORM_BEFORE,
fn (): string => Blade::render('<x-filament::link href="' . config('app.url') . '" size="sm" icon="heroicon-o-arrow-left">Back to Website</x-filament::link>')
);
}
}
Code breakdown
-
Adding Hooks in
AppServiceProvider
:- The
boot()
method in theAppServiceProvider
is ideal for registering hooks globally.
- The
-
Render Hooks Constants:
-
PanelsRenderHook::AUTH_LOGIN_FORM_BEFORE
: Inserts content just before the login form. -
PanelsRenderHook::AUTH_REGISTER_FORM_BEFORE
: Inserts content before the register form.
-
-
Dynamic Links:
- The
config('app.url')
ensures the link dynamically points to the homepage URL defined in the configuration.
- The
-
Blade Rendering:
- The
Blade::render
method allows you to render Blade components or templates directly from PHP.
- The
This pattern is used by Filament Mastery website and you can see it on Login and Registration member pages.
Multiple panels
If your project has multiple panels and you want to apply a render hook to only one of them, you can register it in the corresponding PanelProvider:
use Filament\View\PanelsRenderHook;
use Illuminate\Support\Facades\Blade;
class AdminPanelProvider extends PanelProvider
{
public function panel(Panel $panel): Panel
{
return $panel
...
->renderHook(PanelsRenderHook::AUTH_LOGIN_FORM_BEFORE, fn (): string => Blade::render('<x-filament::link href="' . config('app.url') . '" size="sm" icon="heroicon-o-arrow-left">back to website</x-filament::link>'))
...
}
}
Going further with Render Hooks
Render Hooks are not limited to authentication pages. Filament offers a wide range of Render Hooks for customizing other parts of your panels. For a complete list of available Render Hooks, refer to the Filament Render Hooks documentation.
Render Hooks provide a clean and maintainable way to extend Filament’s functionality. By leveraging these hooks, you can customize your panels while keeping your codebase manageable and future-proof.