· yebor974 · Integration Guides, Plugins & Extensions, Tutorials
Adding terms and conditions acceptance to Filament Registration
Learn how to require acceptance of terms and conditions in your Filament registration page using the filament-terms-guard plugin.
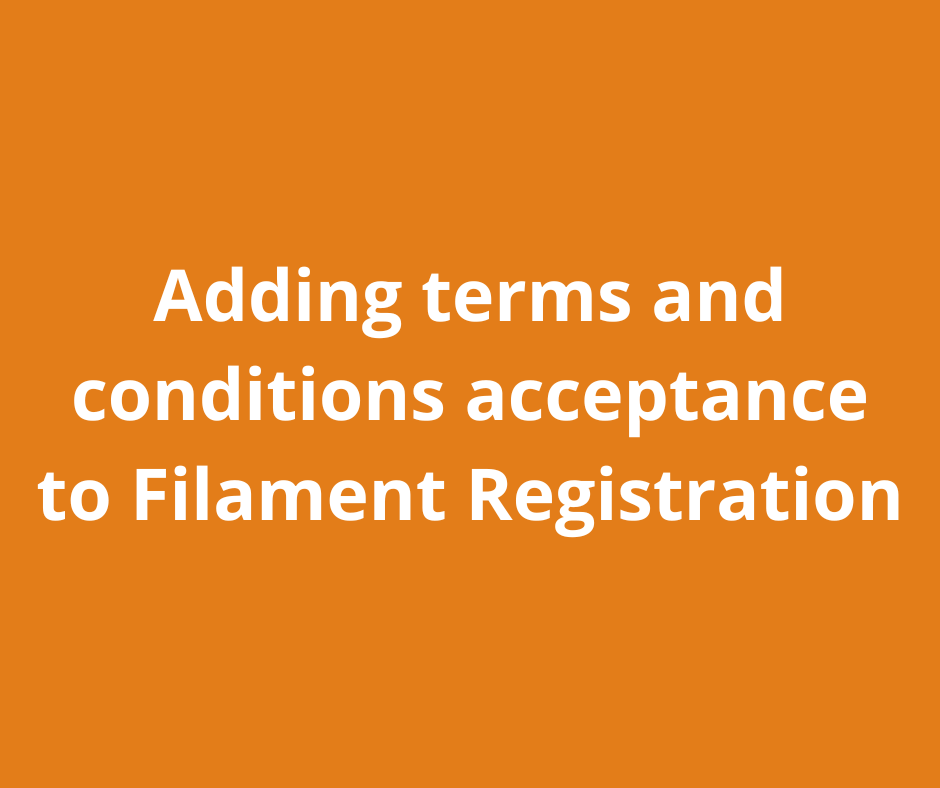
In this tutorial, we'll walk through how to customize a Filament panel’s registration page to include a mandatory checkbox for terms acceptance — using the filament-terms-guard plugin. With this plugin, you can easily create, update, publish, and duplicate terms and conditions, ensuring your users are always up-to-date with the latest policies.
This is especially useful for SaaS dashboards or internal tools that require users to agree to Terms of Service, Privacy Policies, or NDAs before accessing the panel.
1. Installation and setup
Make sure the plugin is installed and registered in your PanelProvider
following the official setup guide.
To activate it for your panel, add this to your .env
file (adjust admin
to match your panel ID):
TERMS_GUARD_PANELS=admin
you can add multiple panels like
admin,member,customer
2. Custom registration page
Instead of using the default Filament registration page, we'll create our own.
Run the following command:
php artisan make:filament-page Register
This creates a page class where we’ll extend the default Filament\Pages\Auth\Register
.
Inside the generated file:
namespace App\Filament\Admin\Pages;
class Register extends \Filament\Pages\Auth\Register
{
// protected static string $view = 'filament.admin.pages.register';
}
Tip: Make sure to comment or remove the
$view
property to keep the default layout.
3. Injecting the terms checkbox
To enforce terms acceptance during registration, we’ll dynamically fetch the current version of the terms published for the current panel, and display a checkbox along with a modal to view them.
The Term::getCurrentTerm() method retrieves the latest published version of the terms for the current panel (admin
in our case). Each panel can have its own set of terms, so this ensures users see the right content depending on where they register.
The terms are stored in a dedicated table and managed via the plugin’s resource. You can publish new versions at any time — the plugin will automatically track user consent against the specific published version.
Here’s how the logic fits together:
- We fetch the current published terms using
Term::getCurrentTerm(panelId)
- The checkbox is required, forcing users to accept before proceeding
- A modal dialog allows users to view the terms inline, with the publication date shown
- Upon successful registration, we associate the accepted version of the terms with the user via
$model->terms()->attach(...)
The updated class looks like this:
namespace App\Filament\Admin\Pages;
use App\Models\User;
use Filament\Forms\Components\Checkbox;
use Filament\Forms\Components\Component;
use Filament\Forms\Components\Actions\Action;
use Filament\Support\Enums\IconSize;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Support\HtmlString;
use Yebor974\FilamentTermsGuard\Models\Term;
class Register extends \Filament\Pages\Auth\Register
{
public Term $terms;
protected function beforeFill(): void
{
$this->terms = Term::getCurrentTerm(filament()->getCurrentPanel()->getId());
}
protected function getForms(): array
{
return [
'form' => $this->form(
$this->makeForm()
->schema([
$this->getNameFormComponent(),
$this->getEmailFormComponent(),
$this->getPasswordFormComponent(),
$this->getPasswordConfirmationFormComponent(),
$this->getTermsFormComponent(),
])
->statePath('data'),
),
];
}
protected function getTermsFormComponent(): Component
{
return Checkbox::make('terms')
->label(fn() => __('filament-terms-guard::terms-guard.actions.accept.submit'))
->hintAction(
Action::make('show-terms')
->icon('heroicon-o-information-circle')
->iconSize(IconSize::Large)
->tooltip('Show terms')
->modalHeading(__('filament-terms-guard::terms-guard.actions.accept.title', [
'date' => $this->terms->published_at->translatedFormat('d F Y')
]))
->modalContent(new HtmlString("<div class='prose max-w-full text-justify'>{$this->terms->content}</div>"))
->modalCancelActionLabel(__('back'))
->modalSubmitAction(false)
)
->accepted();
}
protected function handleRegistration(array $data): Model
{
/** @var User $model */
$model = parent::handleRegistration($data);
$model->terms()->attach($this->terms);
return $model;
}
}
4. Registering the page in your PanelProvider
Finally, update your PanelProvider
to use this custom registration page:
use App\Filament\Admin\Pages\Register;
public function panel(Panel $panel): Panel
{
return $panel
->id('admin')
->login()
->registration(Register::class);
}
Before testing, make sure you’ve created and published a new set of terms for your panel in the database. You can do this via the admin area provided by the plugin or via the model.
Examples
Ready to secure your panel with Terms Guard?
Stop reinventing the wheel. filament-terms-guard handles everything — from terms management to user consent tracking — right out of the box.
👉 Buy the plugin now and get started in minutes.